Instrument Stem Separation Widget Integration
Introduction
AudioShake provides a powerful instrument stem separation tool that can be integrated into your application. This guide covers two integration methods: using the pre-built widget library and using the core JavaScript library for custom implementations.
Widget Library Integration
Including the Script
Add the following script tag to your HTML:
<script src="https://assets.audioshake.ai/audioshake1.1.min.js" type="text/javascript"></script>
Setting Up DOM Elements
Add the following attributes to the button that will trigger the widget:
<td id="sample-element-id-123456"
audioshake-audio-id="sample-song-id-123456789"
audioshake-link="https://link/to/file.wav"
audioshake-filename="ibrahims-songs">Get Stems</td>
Attribute | Description | Example |
---|---|---|
id | A unique id used to present widget popover. On click of this element, the widget popover will present itself next to this element with the given id. | sample-element-id-123456 |
audioshake-audio-id | Unique identifier to represent the ID of the song. | sample-song-id-123456789 |
audioshake-link | HTTP URL that points to a downloadable version of the song for upload. | https://link/to/file.wav |
audioshake-filename | The name of the file for the audio asset. This name will be used to generate the downloadable stems package. | ibrahims-songs |
Instantiating the Widget
function setupAudioShakeWidget() {
const stemOptions = ['vocals', 'instrumental', 'bass', 'drums', 'guitar', 'piano'];
const licenseId = '<licenseId-value>';
const format = 'wav';
var gAudioShakeWidget = new AudioShakeWidgetLib.WidgetPresenter({
licenseId,
format,
stemOptions,
widgetUserSessionId
});
}
The provided configuration parameters needed for setting up the library include:
Field Name | Description |
---|---|
licenseId | A unique Identifier provided by AudioShake to represent the client’s current license to access the widget and API. |
stemOptions | An array of lowercase strings indicating the stem options that the user should be able to choose from. |
format | A lowercase string indicating the output format to generate the stems. Options are: wav, mp3, flac. |
widgetUserSessionId | A Identifier you will need to provide that identifies the user making the request and a valid session that authorizes them to make the request. AudioShake server will use the userSessionId to authenticate with your server to verify this |
Widget Behavior
- Clicking an element with the
audioshake-audio-id
attribute triggers the widget popup. - Users can select stems and initiate generation.
- Progress is displayed during stem generation.
- Once complete, stems can be downloaded as a zip file.
Notes
- Generated stems are available for 24 hours before deletion.
- The widget uses jQuery and Bootstrap, which may interfere with existing dependencies.
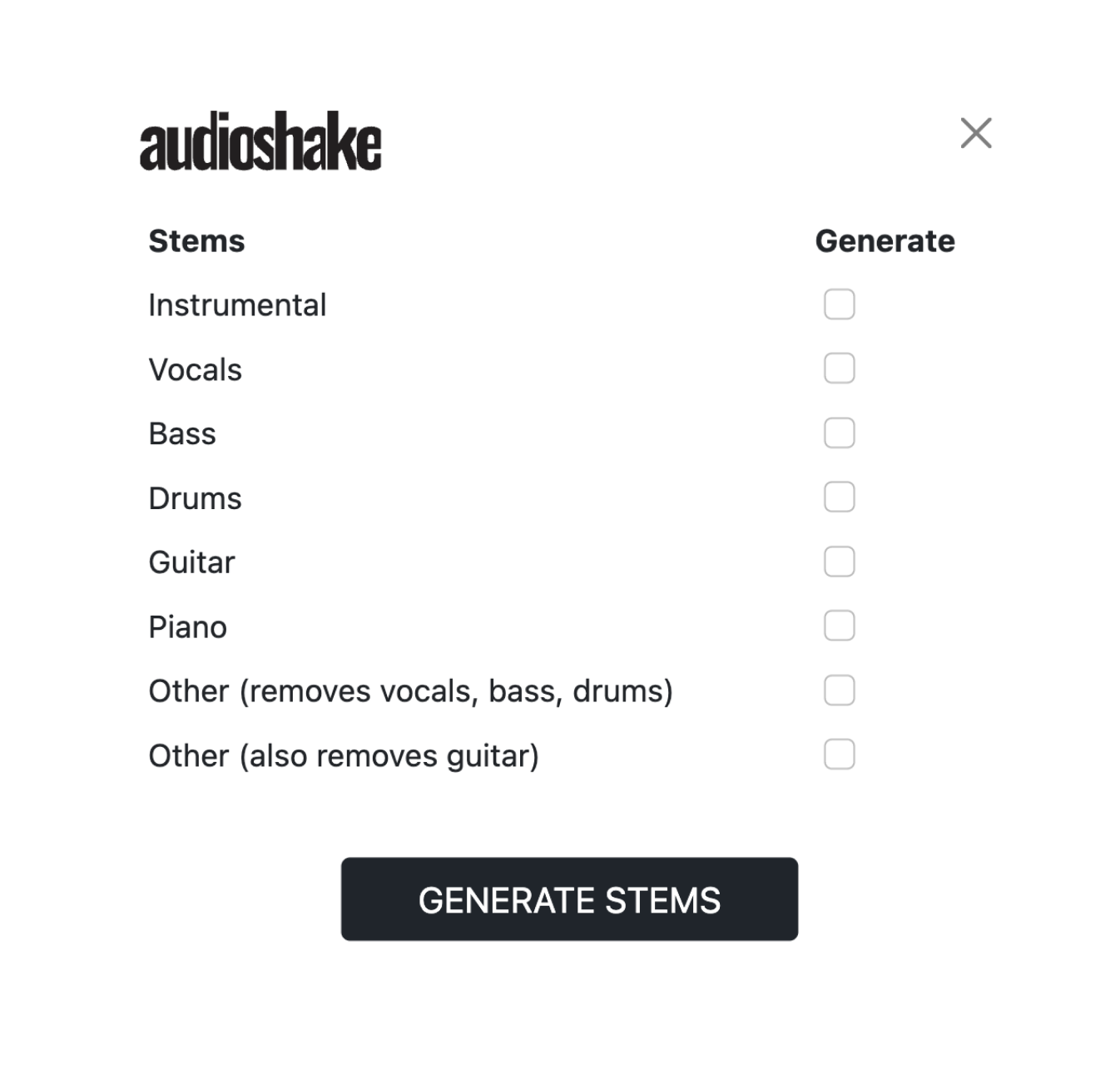
Using AudioShake JS Library without Widget UI
For custom UI implementations, follow these steps:
Instantiating the Core Library
const config = {
audioId,
domain,
licenseId,
onCallback: ({ method, message }) => { console.log(method, message) },
userSessionId
};
const audioShakeCoreLib = new AudioShakeLib.AudioShake(config);
Config Parameter Name | Description |
---|---|
licenseId | (Required) A unique public Identifier provided by AudioShake to represent the client’s current license to access the widget and API. This is NOT an API token |
userSessionId | (Required) An Identifier you will need to provide that identifies the user making the request and a valid session that authorizes them to make the request. AudioShake server will use the userSessionId to authenticate with your server to verify this |
user | user (Read more details below in “Configuring User Authorization” section) |
audioId | (Required) Unique identifier to represent the ID of the song |
domain | (Optional) A domain string of the calling website used to verify web calling host |
onCallback | (Required) A callback to receive messages for different request types. |
The AudioShake core library will immediately try to subscribe to the socket on instantiation. For this reason, we advise that you do not instantiate the library until you know the user is intent on generating stems. This would mean do not open/subscribe to the AudioShake socket until the user clicks on some action button to generate stems.
When the library successfully subscribes to the socket it will send a callback to the 'onCallback' function. The callback will provide a session token and an array of allowed stems for generation as a hint to which model names are allowed when making requests.
NOTE: The core library will attempt to retry the subscription in the case of failure. If after a number of attempts to retry it is not able to connect, then it will send a callback to the 'subscribe' method with a payload indicating that it could not connect to AudioShake servers.
Handling Callbacks
Implement the onCallback
function to handle various events:
onCallback: ({ method, message }) => {
if (message.status === "failed") {
// Handle failures
return
}
if (method === "subscribe") {
// Setup the widget and save the sessionToken for use later. Update UI State
const { sessionToken, allowedModels } = message;
// Once you have sessionToken then it is ok to call generateStems
} else if (method === "create" || method === "status") {
// Retrieve job status and broadcast its current state (processing, failed, complete)
// Update UI state
const jobs = message.jobs;
} else if (method === "create-download-url") {
// Retrieve audioshake download url.
const jobs = message.url;
}
}
Method Name | Message Payload | Explanation |
---|---|---|
All Failed Methods | { status: "failed", description: "Some Description" } | All failed events will have a message payload containing a status as 'failed' and some brief description of what occurred. |
subscribe | { status: ["vocals", "instrumental"], description: "abc" } | Called on successful subscription. 'allowedModels' will contain an array of what stem types are allowed to be generated under this license. 'sessionToken' string will need to be used for all subsequent requests to the library to identify the socket session. |
create | { jobs: [ JobModel ] } | Called immediately after a generateStems request with initial processing status of request stem jobs. Also called when a retryStems. |
status | { jobs: [ JobModel ] } | Called subsequently multiple times as the status changes for each stem job request. All request stem jobs will be included, and for completed jobs, the job will have the appropriate stemAssets which will have links to the stem files. |
create-download-url | { url: ["www.www.www"] } | This will be called after a downloadStems request has been made. The 'url' returned will be a link to a zip package containing the stems. NOTE: this is only necessary if generating more than one stem. |
retry-stems | { status: ["failed"], description: "Some description", jobId: "Job Id" } | When a retry is called, we use the 'create' and 'status' callback methods to send job updates. The 'retry-stems' callback is only an acknowledgment that the retry process was kicked off. |
Generating Stems
function generateStems() {
const stemRequestParams = {
link,
name,
stemNames,
format,
audioId,
userSessionId,
sessionToken,
};
audioShakeCoreLib.generateStems(stemRequestParams);
}
Downloading Stems
Once all of the stems have been generated, the user will then be able to download the stems as a zip file. The stems will be named accordingly based on the name provided in the “audioshake-filename” attribute on the element. For example, an audioshake-filename of "Rainbow Connection" will return as Rainbow Connection_vocal.wav.
function generateDownloadUrl(jobs) {
let links = jobs.map(job => job.stemAssets[0].link);
let fileName = "my stems";
AudioShake.generateDownloadUrl(links, fileName, sessionToken);
}
Disconnecting
You can close the AudioShake socket connection with the 'disconnect' method. This should be called once the user closes the custom widget UI.
function closeWidget() {
audioShakeCoreLib.disconnect();
}
Retrying Stem Generation
You can initiate the retry stems process by calling the 'retryStems' method. This should be called if any stems have failed. Similar to 'generateStems', you should expect that the initial callback to return a 'create' callback payload. All subsequent callbacks being 'status' callbacks.
function resetStems() {
const resetStemsParams = {
audioId,
userSessionId,
sessionToken
};
audioShakeCoreLib.resetStems(resetStemsParams);
}
User Authorization
- Provide AudioShake with a server authorization URL associated with your
licenseId
. - AudioShake will make a POST request to this URL with the
userSessionId
in the JSON body for each stem generation request. - Your server should authenticate the
userSessionId
and authorize the request accordingly.