Lyrics Editor Widget Integration
Introduction
This guide provides instructions for integrating the AudioShake Lyrics Widget into your application.
NOTE: The latest version of lyrics editor widget is 2.4.0.
Important: Before integration, contact AudioShake to whitelist your domain for security reasons.
Below is an example screenshot of the lyrics editor window
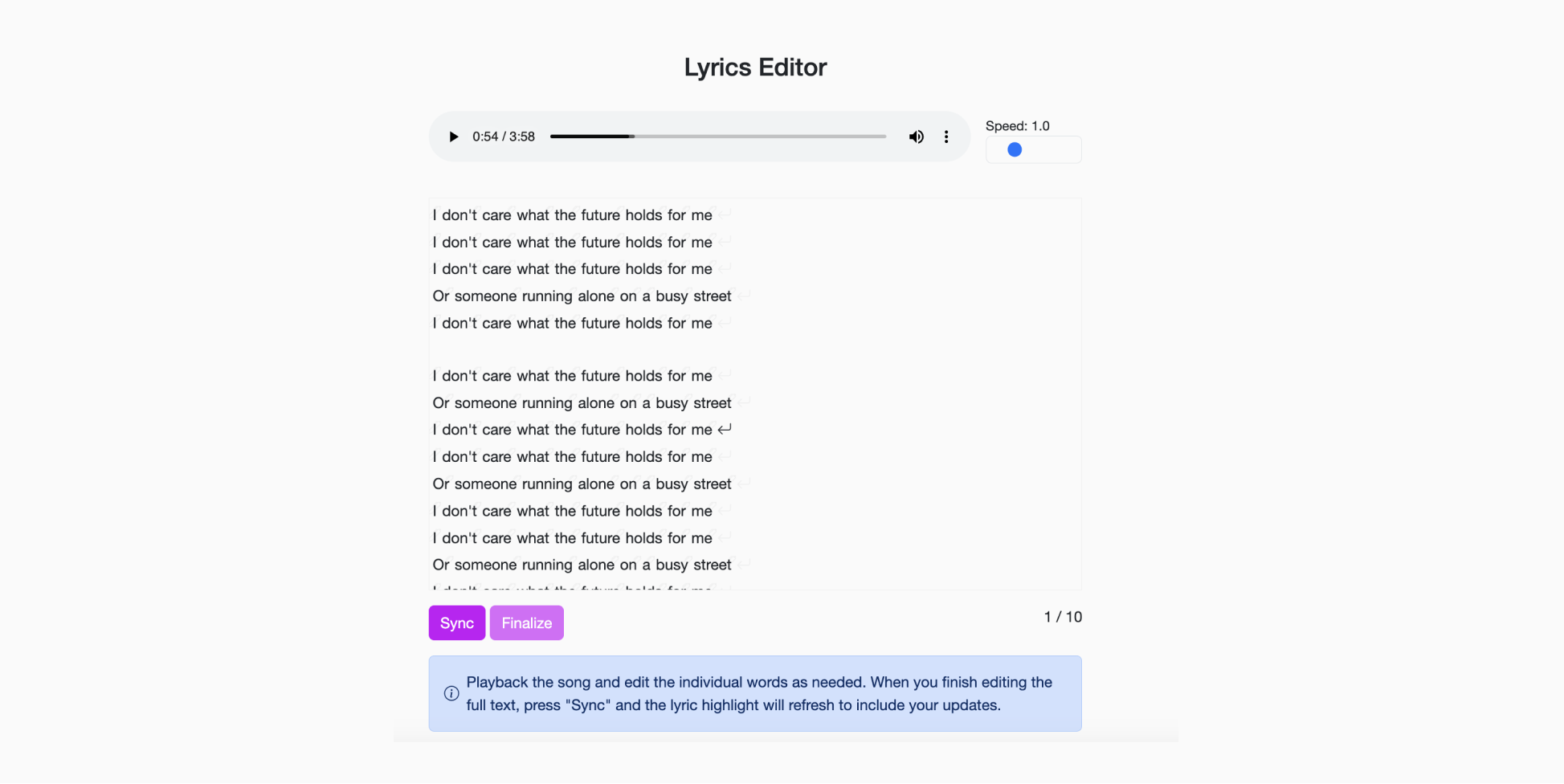
Integration Steps
1. Widget Location
Choose a location in the HTML page where you want to integrate the widget, and then add an <iframe>
tag that will be used to render the widget. You have complete control over where to place the <iframe>
, but you should specify the dimensions of the iframe (e.g. via dynamic CSS styling or HTML height
and width
attributes). You should give the <iframe>
a unique id
that you will use to initialize the widget later. It is recommended to use at least 400px of width and 600px of height.
<iframe height="600px" width="400px" id="lyricsWidget" frameborder="0"></iframe>
2. Library Loading and Initialization
Load the widget library by adding a <script>
tag. Replace the VERSION
with the version that you need. The latest version in indicated in the beginning of this document:
<script src="https://assets.audioshake.ai/widget/lyrics/VERSION/widget.bundle.js"></script>
To initialize the library you need to add the following JavaScript code to either your .js
file or new <script>
tag. The library should be available as a global object AudioShakeLyricsWidgetLib
. It's usually good practice to use something like the DOMContentLoaded
event to run your javacript code:
document.addEventListener('DOMContentLoaded', () => {
const lyricsWidget = new AudioShakeLyricsWidgetLib.LyricsWidget(
licenseId,
userSessionId,
targetIframeId,
maxRequests,
advancedTiming
);
});
Parameter | Description | Example |
---|---|---|
licenseId | Your 25-character license ID | abcde0123456789abcdefghij |
userSessionId | User session ID you generate | user-123 |
targetIframeId | ID of the iframe for rendering | lyricsWidget |
maxRequests (optional) | Maximum sync requests per audio in the session (default: 5) | 10 |
advancedTiming (optional) | Enable advanced timing adjustment, can be 'line' or 'word' | 'line' |
3. Loading Audio
3.1 Interactive Loading
You can reuse the same widget to edit lyrics for a list of tracks. To do that, you should have separate HTML elements that specify the details of individual audio tracks.
Create HTML elements that will load the widget with specific audio track. You can use any html tag, here we show example with <div>
. When the element is clicked, it will load the widget to process the specified audio file:
<div
audioshake-lyrics-widget
audioshake-audio-id="AUDIO_ID"
audioshake-audio-url="PUBLICLY_ACCESSIBLE_AUDIO_URL"
audioshake-transcript-url="PUBLICLY_ACCESSIBLE_TRANSCRIPT_URL"
audioshake-language="fr"
>
Get lyrics
</div>
If you don't need the HTML elements to be interacted to load widget with a particular track, we also provide a method to load the track programmatically:
lyricsWidget.bindLoadingToElements();
3.2 Programmatic Loading
Load tracks programmatically:
lyricsWidget.loadTrack(audioId, audioURL, language, transcriptUrl);
Elements
JS/TS Attribute | DOM Attribute | Description | Example |
---|---|---|---|
N/A | audioshake-lyrics-widget | A required attribute that enables the HTML element to interact with the <iframe> | |
audioId | audioshake-audio-id | A unique id that identifies the track. If the user has already processed a track, the widget will show the result of the previous job for that particular track within the same user session. | abcde0123456789abcdefghij |
audioURL | audioshake-audio-url | A publicly accessible url that should point to the audio track. | https://link/to/audio/file |
language (optional) | audioshake-language (optional) | Specifies the language of the lyrics. It is not necessary, but if known from the metadata, specifying it can increase the accuracy of transcription. It should be a two-letter language code, see the list of supported languages | en |
transcriptUrl (optional) | audioshake-transcript-url (optional) | Public URL of existing transcript for alignment. The transcript should be a JSON file. It can be aligned. | https://link/to/transcript/file |
4. Receiving the Aligned Transcript
Register a callback to receive the final result:
lyricsWidget.registerOnResultReceived((result) => {
const { audioId, licenseId, userSessionId, audioUrl, alignedTranscriptUrl, assets } = result;
// Handle the result
});
Element | Description | Examples |
---|---|---|
audioId | The ID of the audio file | 1234567890abcdef |
licenseId | Your 25-char license ID | ABCDEF1234567890ABCDEF1234 |
userSessionId | User session id that you generate. Transcribed lyrics are cached within the session, and maxRequest limit applies | sess-1234abcd5678efgh |
audioUrl | A download link to the audio file | https://example.com/audio/1234567890abcdef.mp3 |
alignedTranscriptUrl | A download link to the aligned transcript | https://example.com/transcript/1234567890abcdef.txt |
assets | Provides the resulting transcript in several formats (e.g .txt , .srt ) | [ { "name": "transcript.txt", "id": "cm13dp87g0003otmj5rpr5otxt", "fileType": "text/plain", "format": "txt", "link": "https://example.com/transcript.txt" }, { "name": "transcript.json", "id": "cm13dp87g0003otmj5rpr5ojson", "fileType": "application/json", "format": "json", "link": "https://example.com/transcript.json" }, { "name": "alignment.srt", "id": "cm13dp87g0003otmj5rpr5osrt", "fileType": "application/x-subrip", "format": "srt", "link": "https://example.com/alignment.srt" } ] |
5. Receiving Intermediate Results (Optional)
Register a callback for intermediate results:
lyricsWidget.registerOnIntermediateResultReceived((result) => {
const { audioId, licenseId, userSessionId, audioUrl, alignedTranscriptUrl, assets } = result;
// Handle intermediate result
});
6. Handling Errors (Optional)
Register an error callback:
lyricsWidget.registerOnErrorReceived((error) => {
const { code, message } = error;
// Handle the error
});
7. Widget Customization (Optional)
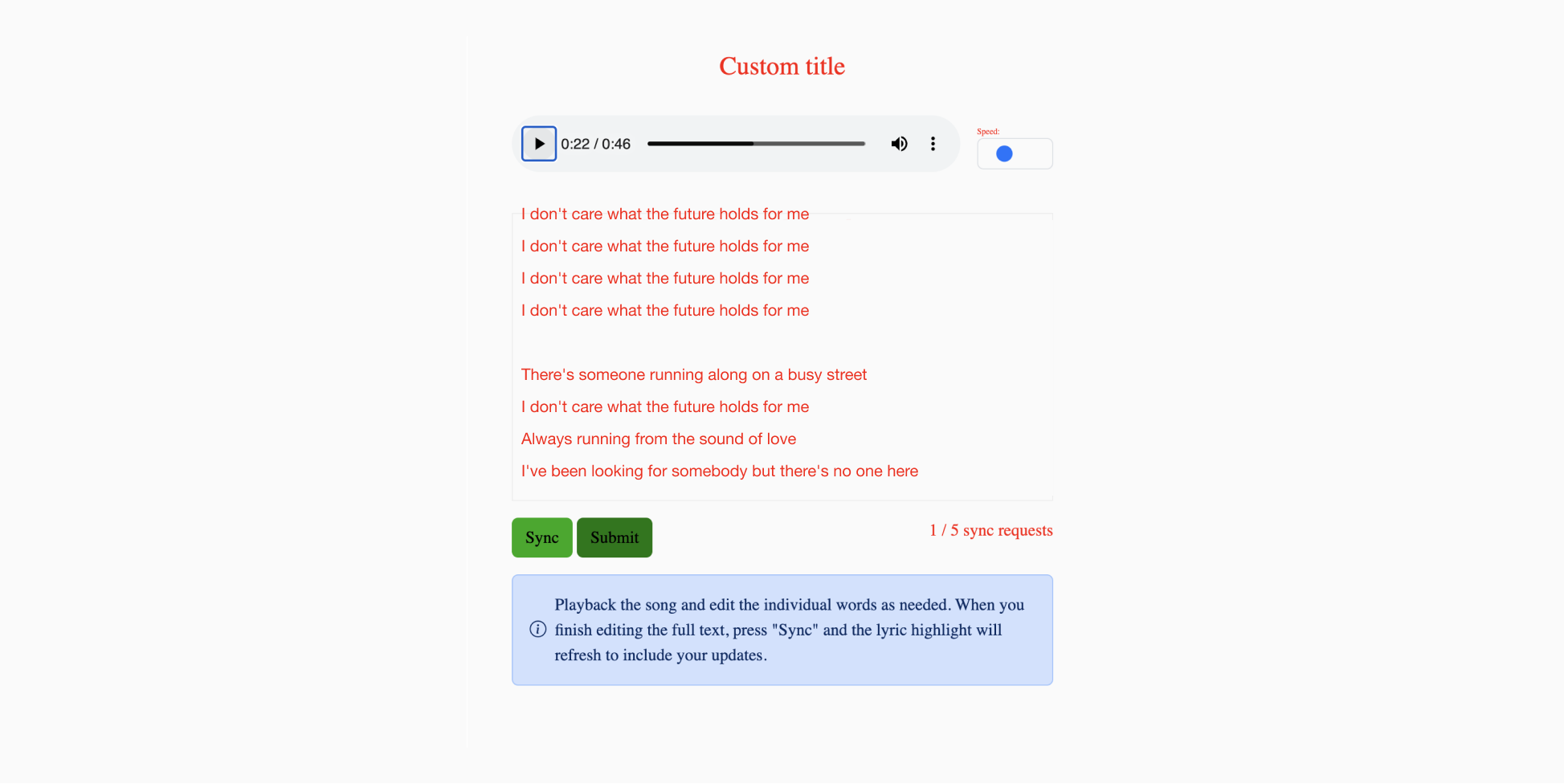
After initialization of the lyricsWidget
, you can also call .customize
to customize widget style and some content:
lyricsWidget.customize({
title: 'Custom title',
fontFamily: 'Times',
colors: {
background: '#FAFAFA',
text: 'red',
lyricsProgress: 'yellow', // the progress bar on lyrics during playback
primary: {
// buttons and other interactable elements
regular: '#00AA00', // regular color
hover: '#007700', // color change when hovering
text: 'black', // text color (to maintain contrast if necessary)
},
},
});
Almost every field in customization object is optional, so you can customize only the things that you need, e.g.
lyricsWidget.customize({
colors: {
background: "green",
}
}
Customization options:
type CustomizationBtn = {
regular: string;
hover?: string;
text?: string;
};
type Customization = {
title?: string;
fontFamily?: string;
colors?: {
background?: string;
text?: string;
lyricsProgress?: string;
primary?: CustomizationBtn;
};
};
For any issues or questions, please contact support@audioshake.ai.